BiConsumer Functional Interface in Java
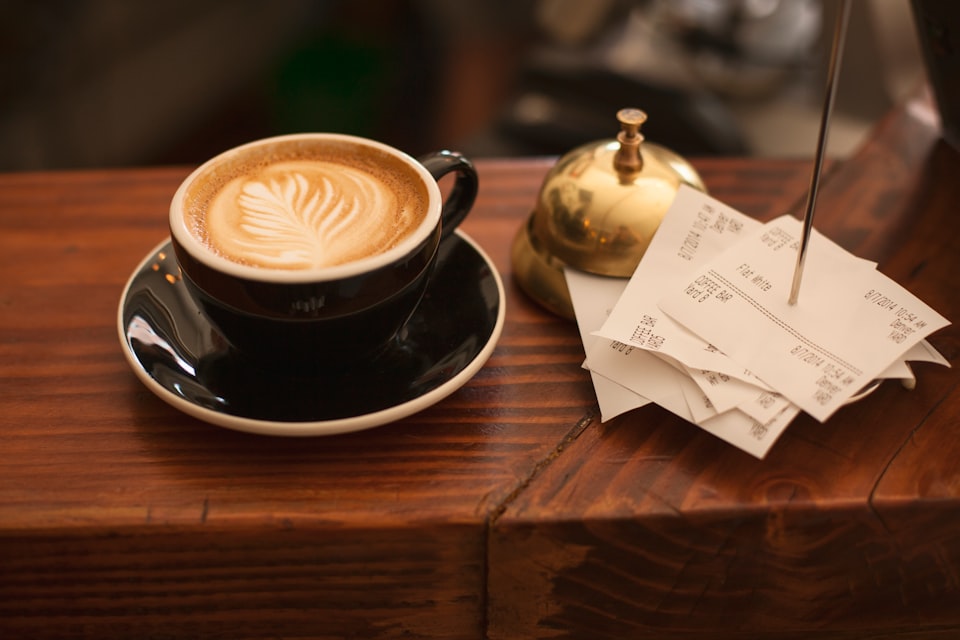
In the previous post, we discussed Functional Interfaces. Here, we will discuss the BiConsumer Functional interface, which derives from the Base Functional Interface- Consumer, based on the number of arguments it takes; two in this case.
The structure of the BiConsumer Functional Interface is shown below. It accepts two input arguments instead of one, and does not return a value. Recall that just like Consumer, and unlike most other Functional interfaces, the BiConsumer works via side-effects. They are super useful when you are not expected to return a result.
public interface BiConsumer <T,U> {
void accept(T t, U u);
// omitted default andThen method
}
BiConsumer Examples
- Let's assume your application reads rows from a CSV file, and it has two columns for phone number Country code and another for the actual telephone number. You are unsure whether the telephone numbers are in the international format. Your task is simply to read each CSV row and print a single international phone number.
import java.util.function.BiConsumer;
public class BiConsumerExample {
public static void main(String[] args) {
BiConsumer parsePhoneNumbers = (countryCode, phoneNumber) -> {
String formattedPhoneNumber = phoneNumber.startsWith(countryCode) ? phoneNumber : countryCode.concat(phoneNumber);
System.out.println(formattedPhoneNumber);
};
parsePhoneNumbers.accept("+234", "07031925674");
parsePhoneNumbers.accept("+49", "892938232948");
parsePhoneNumbers.accept("+49", "887299230244");
parsePhoneNumbers.accept("+234", "+23408031925675");
}
}
Line 7 declares the BiConsumer parsePhoneNumbers, which receives as input the countryCode and the phoneNumber. In the BiConsumer function, we simply check if the phoneNumber already starts with the country code; meaning it's already internationalized. If it is, we leave it as is, otherwise, we internationalize it by placing the country code in front of it. And then we proceed to print the internationalized phone number.
Figure 1 shows the result
- You could also write a BiConsumer to perform a function on two generic input arguments. You can then write lambda expressions for each given case, and pass the lambda to this BiConsumer, stating how it should do what you asked. This is pure power. See the example below
import java.util.function.BiConsumer;
public class BiConsumerExample {
public static void main(String[] args) {
processTwoValues(3, 5, (x,y) -> System.out.println(x + "+" + y +"= " + (x + y)));
processTwoValues("The chicken crossed the road", "Ali goes to School", (sentence1, sentence2) -> {
System.out.println("Words in both Sentences: " + (sentence1.split("\\w+").length + sentence2.split("\\w+").length));
});
}
static <T> void processTwoValues(T t1, T t2, BiConsumer biConsumer) {
biConsumer.accept(t1, t2);
}
}
Figure 2 shows the result
Happy Learning!!!
Member discussion