Abstract classes and methods in Java
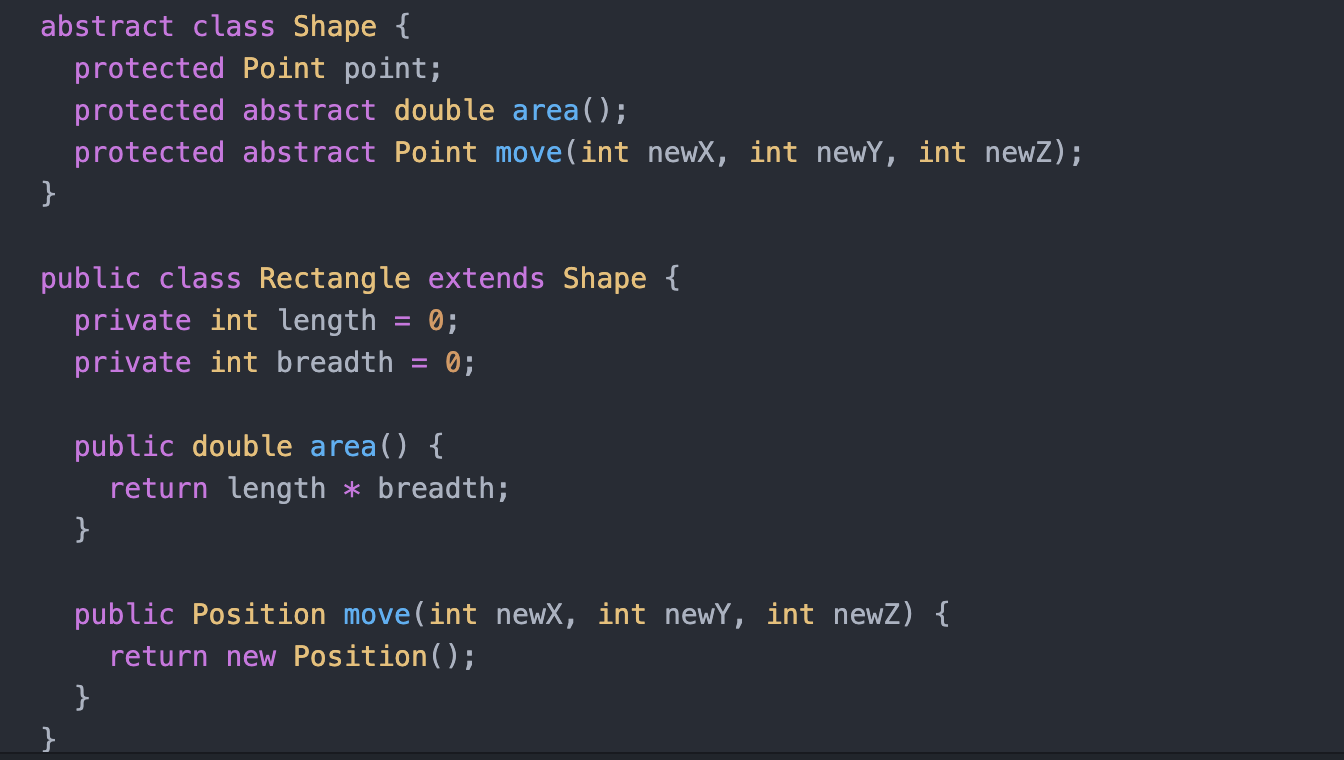
An abstract class, similar to an interface serves as a base to create subclasses. It cannot be instantiated, may or may not contain an abstract method.
package card;
import card.enumeration.Orientation;
import card.enumeration.CardFace;
import card.enumeration.CardType;
public abstract class Card {
protected String serial;
protected String qrCodeImageUrl;
protected CardAttribute cardAttribute;
public String getQrCodeImageUrl() {return this.qrCodeImageUrl;}
public String getCardSerial() {return this.cardSerial;}
}
The snippet above is an abstract class containing fields and methods. Notice it does not need to have an abstract method.
Points to note about abstract classes and methods
- An abstract class is one declared with the keyword abstract
- An abstract class can be declared without an implementation.
- An abstract class may contain any number of methods, including zero.
- Methods in an abstract class can be concrete or abstract.
- An abstract class may or may not contain abstract methods
- An abstract class can be subclassed
- An abstract class can contain fields that are non static and final
- Public, protected and private concrete methods can be declared in an abstract class.
- All methods in an abstract class, declared as default are implictly public
- An abstract methods may not appear in a class that is not abstract.
- The first concrete sublass of an abstract class is required to implement all abstract methods that were not implemented in a superclass
Member discussion